HP ALM REST API - Authentication
. 3 mins read
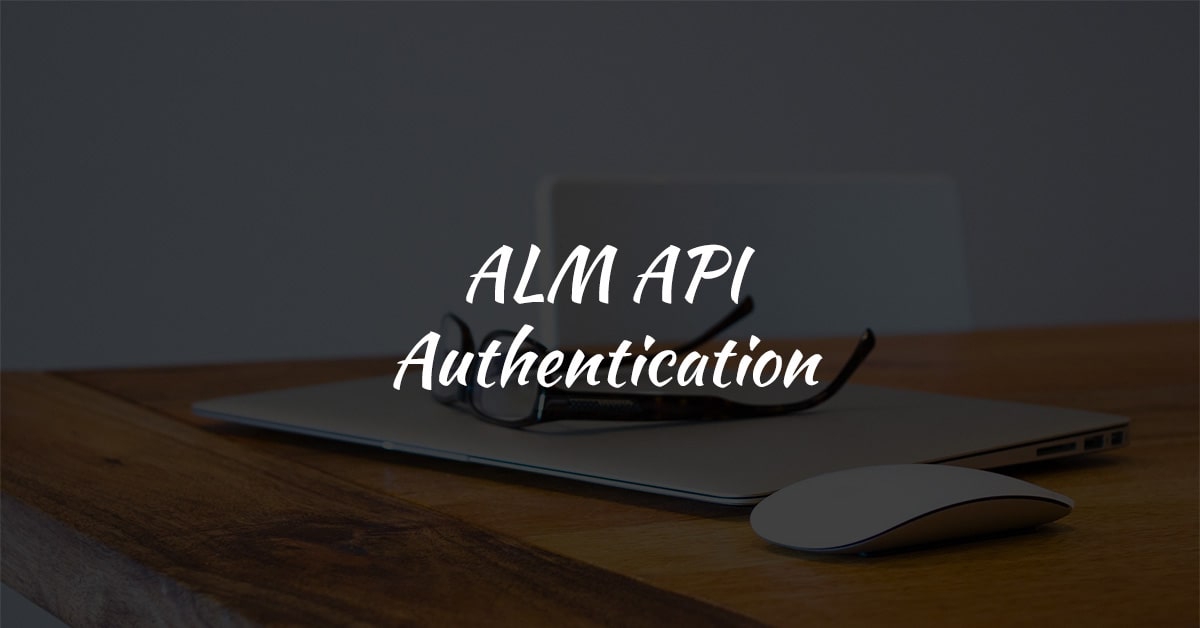
Tutorial on how to authenticate HP ALM Session using REST API. Includes VB.NET and C# code for Sign-in and Sign-out resources.
HP ALM provides two resources for Authentication.
- Sign-in
- Sign-out
Sign-in
The sign-in resource request authorization. To successfully authenticate we have to post user and password in BASE64 encoding in the Authorization header.
Sign-in resource sets the authentication cookies required for future requests.
- ALM_USER
- LWSSO_COOKIE_KEY
- QCSession
- XSRF-TOKEN
Sign-in URI
Uri for sign-in is /qcbin/api/authentication/sign-in
Example http://youralmserver:port/qcbin/api/authentication/sign-in
For ALM Versions prior to 12.53, uri for sign-in is
/qcbin/authentication-point/authenticate?login-form-required=y
Example
http://youralmserver:port/qcbin/authentication-point/authenticate?login-form-required=y
If this URI is provided directly to the browser, you will get a prompt to enter your user name and password. Once you enter the correct credentials you will be taken to a blank screen.
Doing it with code
Parameters Required
Parameter | Example |
---|---|
HP ALM Base URL | http://youralmurl:port/qcbin |
HP ALM User Name | userName |
HP ALM Password | Password |
Sample Response
**** Request ****
POST /qcbin/api/authentication/sign-in HTTP/1.1
Authorization: Basic 123456789abcdef=
Host: myserver:8081
**** Response ****
HTTP/1.1 200 OK
Set-Cookie: LWSSO_COOKIE_KEY=0123456789abcdef;Path=/;HTTPOnly
Set-Cookie: QCSession=0123456789abcdef;Path=/;HttpOnly
Expires: Thu, 01 Jan 1970 00:00:00 GMT
Set-Cookie: ALM_USER=0123456789abcdef;Path=/
Set-Cookie: XSRF-TOKEN=0123456789abcdef;Path=/
Content-Length: 0
Sign-in Code
VB.NET code to get Authentication Cookies
Private Function GetAuthenticationCookieContainer(almBaseUrl, almUserName, almPassword) As CookieContainer
Dim authCookies = new CookieContainer()
Dim auth As HttpWebRequest = WebRequest.Create(almBaseUrl + "/api/authentication/sign-in")
Dim credentials As String = $"{almUserName}:{almPassword}"
auth.CookieContainer = authCookies
auth.Headers.Set(HttpRequestHeader.Authorization, "Basic " + Convert.ToBase64String(Encoding.UTF8.GetBytes(credentials)))
Dim authResult As WebResponse = auth.GetResponse()
Return authCookies
End Function
Using VB code
Dim AuthenticationCookieContainer = GetAuthenticationCookieContainer("http://youralmurl:port/qcbin", "aneejian", "Password")
C# code to get Authentication Cookies
private static CookieContainer GetAuthenticationCookie(string almBaseUrl, string almUserName, string almPassword)
{
var authCookies = new CookieContainer();
var auth = (HttpWebRequest)WebRequest.Create(almBaseUrl + "/api/authentication/sign-in");
var credentials = $"{almUserName}:{almPassword}";
auth.CookieContainer = authCookies;
auth.Headers.Set(HttpRequestHeader.Authorization, "Basic " + Convert.ToBase64String(Encoding.UTF8.GetBytes(credentials)));
var authResult = auth.GetResponse();
return authCookies;
}
Using C# Code
var authenticationCookieContainer = GetAuthenticationCookie("http://youralmurl:port/qcbin", "aneejian", "Password");
For ALM Versions prior to 12.53, in the code replace
/api/authentication/sign-in
with/authentication-point/authenticate
.
Sign-out
The sign-out resource logs the user off the system. It cancels the cookies returned by Sign-in.
- LWSSO_COOKIE_KEY
- QCSession
- ALM_USER
- XSRF-TOKEN
Sign-out URI
Uri for sign-out is /qcbin/api/authentication/sign-out
Example http://youralmserver:port/qcbin/api/authentication/sign-out
For ALM Versions prior to 12.53, uri for sign-out is
/qcbin/authentication-point/logout
Example
http://youralmserver:port/qcbin/authentication-point/logout
If you pass this to the browser where you signed in, it will sign you out from ALM.
Sign-out Code
VB.NET code to Sign-out
Private Sub Signout(almBaseUrl As String, AuthenticationCookieContainer)
Dim auth = CType(WebRequest.Create(almBaseUrl + "/api/authentication/sign-out"), HttpWebRequest)
auth.CookieContainer = AuthenticationCookieContainer
Dim authResult = auth.GetResponse()
End Sub
Usage of VB code to Sign-out
SignOut("http://youralmurl:port/qcbin", AuthenticationCookieContainer)
C# code to Sign-out
private static void SignOut(string almBaseUrl, CookieContainer authenticationCookieContainer)
{
var auth = (HttpWebRequest)WebRequest.Create(almBaseUrl + "/api/authentication/sign-out");
auth.CookieContainer = authenticationCookieContainer; //authenticationCookieContainer is the CookieContainer you got from GetAuthenticationCookieContainer function.
var authResult = auth.GetResponse();
}
Usage of C# code to Sign-out
SignOut("http://youralmurl:port/qcbin", authenticationCookieContainer);
For ALM Versions prior to 12.53, in the code replace
/authentication/sign-out
with/authentication-point/logout
- Programming
- Software Testing
Categories: