HP ALM REST API - Get ALM Projects
. 3 mins read
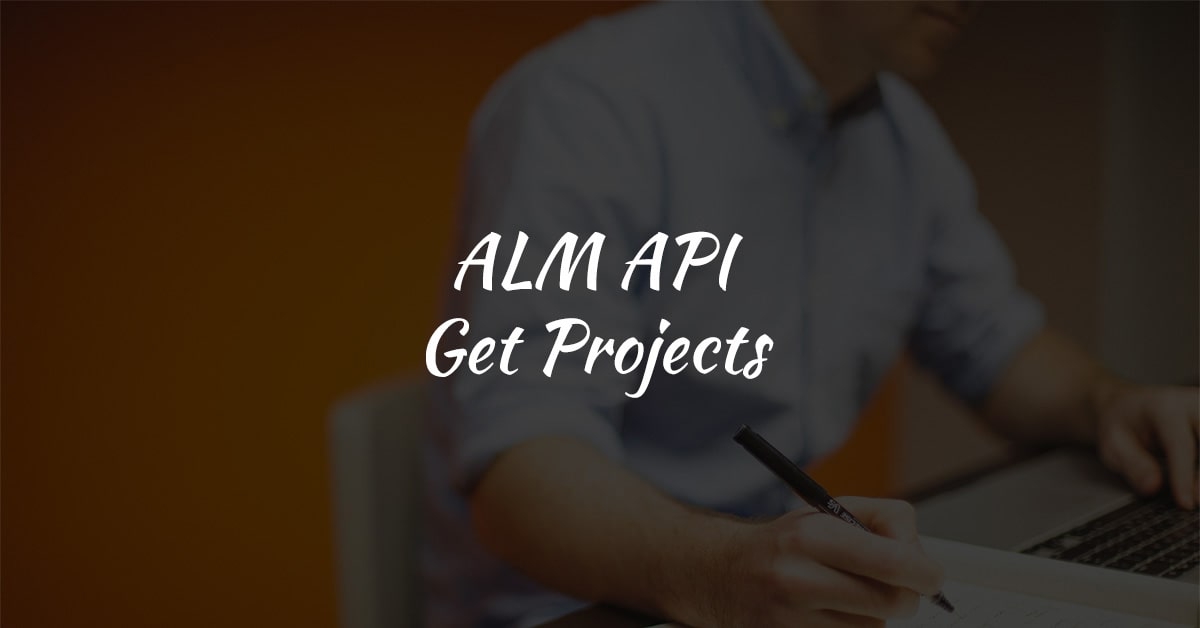
Tutorial on how to get projects from HP ALM using REST API. Includes the browser method, VB.NET and C# functions to get domains.
Web Browser Method
- Open your favorite browser.
- Enter HP ALM REST API URL:
http://youralmserver:port/qcbin/api/authentication/sign-in
- Enter your ALM User Name and Password in the prompt that you get on navigating to the above URL.
- Once successful authentication has occurred you will get a blank page.
- Now enter the URL to get domains in the same browser window:
http://youralmserver:port/qcbin/api/domains/domainName/projects
(domainName is the name of the domain whose projects you want to view. A domain name is case sensitive. Use the name as displayed in ALM.) - Once you enter this URL and press enter you will get the list of projects you have access to under the domain in XML format.
For ALM Versions prior to 12.53, in URLs, replace
api
withrest
Example
http://youralmserver:port/qcbin/rest/domains/domainName/projects
Refer HP ALM REST API - Authentication for details on how to sign-in in older versions of ALM.
Sample Output
<Projects>
<Project UID="172a-321-543-1" Name="Project1" />
<Project UID="172a-321-543-2" Name="Project2" />
<Project UID="172a-321-543-3" Name="Project3" />
<Project UID="172a-321-543-4" Name="Project4" />
<Project UID="172a-321-543-5" Name="Project5" />
</Projects>
Code Method
Parameters Required
Parameter | Example / Comments |
---|---|
HP ALM Base URL | http://youralmurl:port/qcbin |
HP ALM Domain Name | Enter the name of the domain from which you want the list of projects. Refer HP ALM REST API - Get ALM Domains for details on how to get domains from ALM. |
Authentication CookieContainer | Pass the authentication cookie container obtained while you authenticated. Refer HP ALM REST API - Authentication for details. |
For ALM Versions prior to 12.53, in the code replace
/api/domains
with/rest/domains
.
VB.NET Code to get ALM Projects
Private Function GetProjects(almBaseUrl As String, domainName As String, authenticationCookieContainer As CookieContainer) As IEnumerable(Of String)
Dim projectList = New List(Of String)()
Dim projectRestApiUrl = $"{almBaseUrl}/api/domains/{domainName}/projects"
Dim projectRequest = CType(WebRequest.Create(projectRestApiUrl), HttpWebRequest)
projectRequest.CookieContainer = authenticationCookieContainer
Dim projectRequestResponse = projectRequest.GetResponse()
Dim projectRequestResponseStream = projectRequestResponse.GetResponseStream()
If projectRequestResponseStream Is Nothing Then
Return projectList
End If
Using responseReader = New StreamReader(projectRequestResponseStream)
Dim responseString = responseReader.ReadToEnd()
Dim responseXml = XElement.Parse(responseString)
Dim projectQuery = From project In responseXml.Elements() Select New With {.projectName = project.Attribute("Name").Value}
projectList.AddRange(projectQuery.[Select](Function(project) project.projectName))
End Using
Return projectList
End Function
Usage of VB.NET code
Dim almProjects = GetProjects("http://youralmurl:port/qcbin", "Domain1", AuthenticationCookieContainer)
Console.WriteLine(almProjects.Aggregate(Function(a, b) a + vbLf + b))
C# Code to get ALM Projects
private static IEnumerable GetProjects(string almBaseUrl, string domainName, CookieContainer authenticationCookieContainer)
{
var projectList = new List();
var projectRestApiUrl = $"{almBaseUrl}/api/domains/{domainName}/projects";
var projectRequest = (HttpWebRequest)WebRequest.Create(projectRestApiUrl);
projectRequest.CookieContainer = authenticationCookieContainer;
var projectRequestResponse = projectRequest.GetResponse();
var projectRequestResponseStream = projectRequestResponse.GetResponseStream();
if (projectRequestResponseStream == null) return projectList;
using (var responseReader = new StreamReader(projectRequestResponseStream))
{
var responseString = responseReader.ReadToEnd();
var responseXml = XElement.Parse(responseString);
var projectQuery = from project in responseXml.Elements()
select new { projectName = project.Attribute("Name").Value };
projectList.AddRange(projectQuery.Select(project => project.projectName));
}
return projectList;
}
Usage of C# code
var almProjects = GetProjects("http://youralmurl:port/qcbin", "Domain1", authenticationCookieContainer);
Console.WriteLine(almProjects.Aggregate((a, b) => a + "\n" + b));
Output Sample
Project1
Project2
Project3
Project4
Project5
- Programming
- Software Testing
Categories: