HP ALM REST API - Get ALM Domains and Projects Together
. 4 mins read
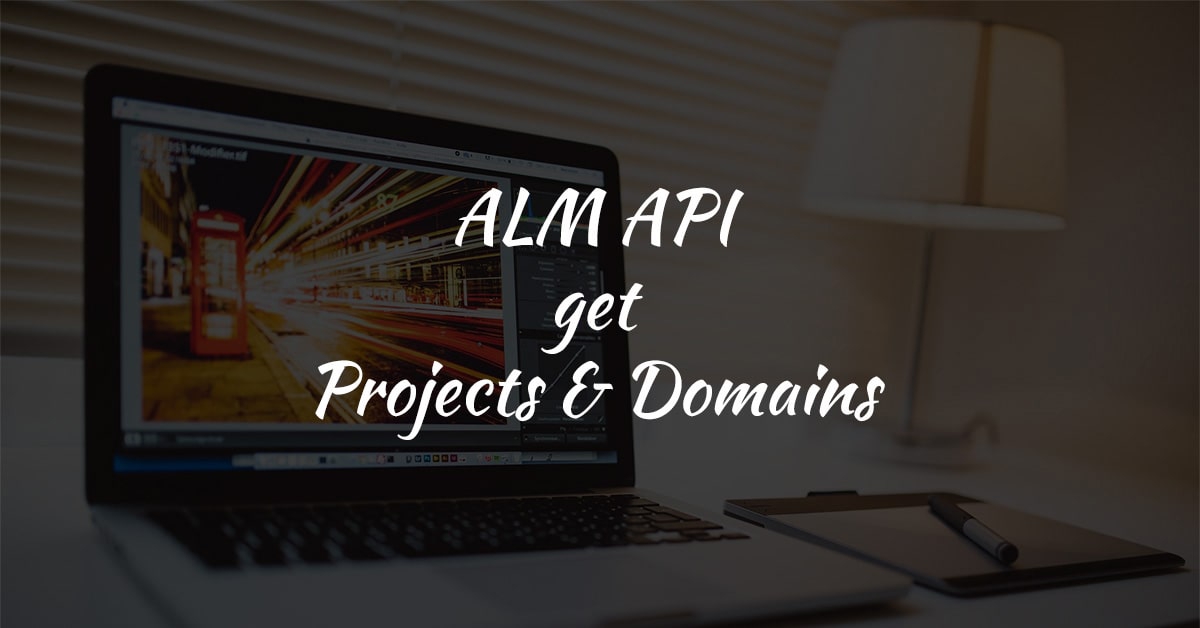
Tutorial on how to get domains and projects from HP ALM using REST API together. Includes browser method, VB.NET and C# functions to get them together.
Web Browser Method
- Open your favorite browser.
- Enter HP ALM REST API URL:
http://youralmserver:port/qcbin/api/authentication/sign-in
- Enter your ALM User Name and Password in the prompt that you get on navigating to the above URL.
- Once successful authentication has occurred you will get a blank page.
- Now enter the URL to get domains in the same browser window:
http://youralmserver:port/qcbin/api/domains?include-projects-info=y
- Once you enter this URL and press enter you will get the list of domains and projects under them. Resulting XML includes all domains and projects you have access to in HP ALM.
For ALM Versions prior to 12.53, in URLs, replace
api
withrest
e.g.
http://youralmserver:port/qcbin/rest/domains?include-projects-info=y
Refer HP ALM REST API - Authentication for details on how to sign-in in older versions of ALM.
Sample Output
<Domains>
<Domain Name="Domain1">
<Projects>
<Project UID="172a-321-543-1" Name="Project1" />
<Project UID="172a-321-543-2" Name="Project2" />
<Project UID="172a-321-543-3" Name="Project3" />
</Projects>
</Domain>
<Domain Name="Domain2">
<Projects>
<Project UID="172a-321-543-11" Name="ProjectA" />
<Project UID="172a-321-543-21" Name="ProjectB" />
<Project UID="172a-321-543-43" Name="ProjectC" />
<Project UID="172a-321-543-44" Name="ProjectD" />
<Project UID="172a-321-543-56" Name="ProjectE" />
</Projects>
</Domain>
<Domain Name="Domain3">
<Projects>
<Project UID="172a-321-543-31" Name="ProjectX" />
<Project UID="172a-321-543-25" Name="ProjectY" />
</Projects>
</Domain>
</Domains>
Code Method
Required Parameters
Parameter | Example / Comments |
---|---|
HP ALM Base URL | http://youralmurl:port/qcbin |
Authentication CookieContainer | Pass the authentication cookie container obtained while you authenticated. Refer HP ALM REST API - Authentication for details. |
In order to store Release information, create a class ProjectReleases
Refer respective code sections for the class definition
For ALM Versions prior to 12.53
In the code replace /api/domains
with /rest/domains
VB.NET Code
VB.NET Code to define DomainProject
class
Friend Class DomainProject
Public Property Domain() As String
Get
Return m_Domain
End Get
Set
m_Domain = Value
End Set
End Property
Private m_Domain As String
Public Property ProjectList As List(Of String) = New List(Of String)
Public Overrides Function ToString() As String
Return $"Domain: {Domain}{vbLf}Project Count: {ProjectList.Count}{vbLf}{vbTab}{ProjectList.Aggregate(Function(a,b) a + vbLf + vbTab + b)}{vbLf}"
End Function
End Class
VB.NET Code to get ALM Domains and Projects Together
Private Function GetDomainProject(almBaseUrl As String, authenticationCookieContainer As CookieContainer) As List(Of DomainProject)
Dim domainProjectList = New List(Of DomainProject)()
Dim domainProjectRequest = CType(WebRequest.Create(almBaseUrl + "/api/domains?include-projects-info=y"), HttpWebRequest)
domainProjectRequest.CookieContainer = authenticationCookieContainer
Dim domainProjectRequestResponse = domainProjectRequest.GetResponse()
Dim domainProjectRequestResponseStream = domainProjectRequestResponse.GetResponseStream()
If domainProjectRequestResponseStream Is Nothing Then
Return domainProjectList
End If
Using responseReader = New StreamReader(domainProjectRequestResponseStream)
Dim responseString = responseReader.ReadToEnd()
Dim responseXml = XElement.Parse(responseString)
Dim projectListXml = responseXml.Descendants("Projects")
Dim projectQuery = From project In projectListXml.Elements()
Let domainName = project.Parent.Parent.FirstAttribute.Value
Let projectName = project.Attribute("Name").Value
Order By domainName, projectName
Select New With {domainName, projectName}
domainProjectList = projectQuery.GroupBy(Function(d) d.domainName).[Select](Function(p) New DomainProject With {.Domain = p.Key, .ProjectList = p.[Select](Function(q) q.projectName).ToList()}).ToList()
End Using
Return domainProjectList
End Function
Usage of VB.NET code
Dim almDomainProjects = GetDomainProject(almBaseUrl, authenticationCookieContainer);
For Each dp As DomainProject in almDomainProjects
Console.WriteLine(dp.ToString());
Next
C# Code
C# Code to define DomainProject
class
internal class DomainProject
{
public string Domain { get; set; }
public List ProjectList { get; set; }
public override string ToString()
{
return $"Domain: {Domain} \nProject Count: {ProjectList.Count} \n\t{ProjectList.Aggregate((a, b) => a + "\n\t" + b)}\n";
}
}
C# Code to get ALM Domains and Projects Together
private static IEnumerable GetDomainProject(string almBaseUrl, CookieContainer authenticationCookieContainer)
{
var domainProjectList = new List();
var domainProjectRequest = (HttpWebRequest)WebRequest.Create(almBaseUrl + "/api/domains?include-projects-info=y");
domainProjectRequest.CookieContainer = authenticationCookieContainer;
var domainProjectRequestResponse = domainProjectRequest.GetResponse();
var domainProjectRequestResponseStream = domainProjectRequestResponse.GetResponseStream();
if (domainProjectRequestResponseStream == null) return domainProjectList;
using (var responseReader = new StreamReader(domainProjectRequestResponseStream))
{
var responseString = responseReader.ReadToEnd();
var responseXml = XElement.Parse(responseString);
var projectListXml = responseXml.Descendants("Projects");
var projectQuery = from project in projectListXml.Elements()
let domainName = project.Parent.Parent.FirstAttribute.Value
let projectName = project.Attribute("Name").Value
orderby domainName, projectName
select new { domainName, projectName };
domainProjectList = (from d in projectQuery group d.projectName by d.domainName into dp select new DomainProject { Domain = dp.Key, ProjectList = dp.ToList() }).ToList();
}
return domainProjectList;
}
Usage of C# code
var almDomainProjects = GetDomainProject(almBaseUrl, authenticationCookieContainer);
foreach (var dp in almDomainProjects)
{
Console.WriteLine(dp.ToString());
}
Output sample
Domain: Domain1
Project Count: 3
Project1
Project2
Project3
Domain: Domain2
Project Count: 5
ProjectA
ProjectB
ProjectC
ProjectD
ProjectE
Domain: Domain3
Project Count: 2
ProjectX
ProjectY
You can use this code to get information from all domains and projects without hard coding domain names and project names. Use the DomainProject
class to get data from various Domain-Project combinations.
- Programming
- Software Testing
Categories: