HP ALM REST API - Get ALM Domains
. 3 mins read
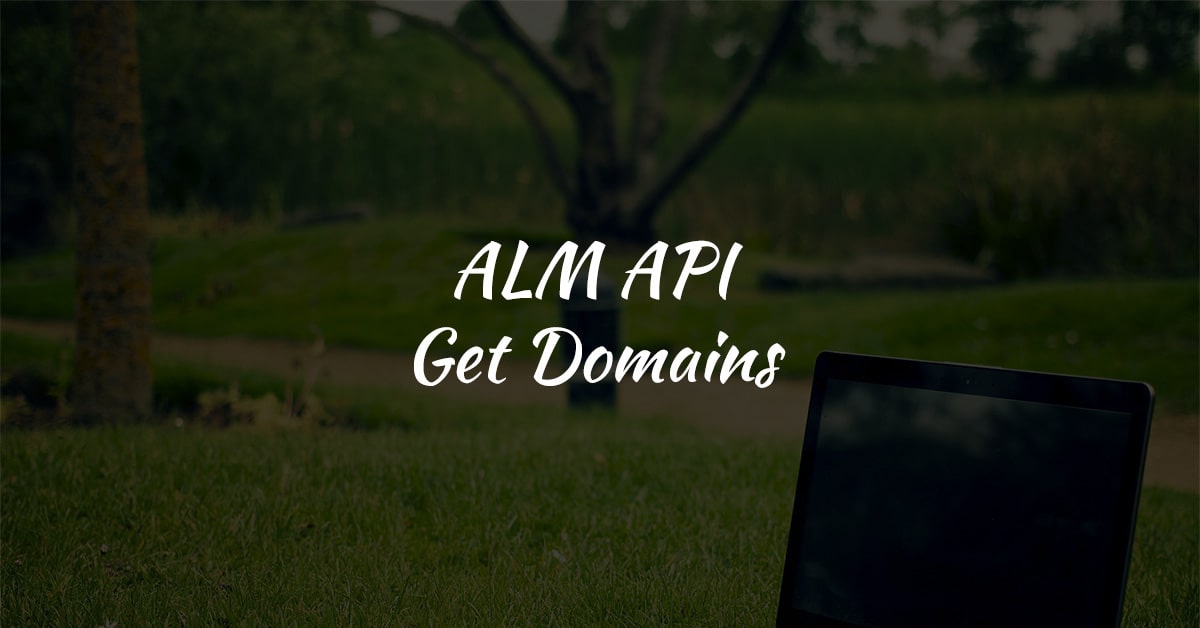
Tutorial on how to get domains from HP ALM using REST API. Includes the browser method, VB.NET and C# functions to get domains. Learn more with Aneejian.
Web Browser Method
- Open your favorite browser.
- Enter HP ALM REST API URL:
http://youralmserver:port/qcbin/api/authentication/sign-in
- Enter your ALM User Name and Password in the prompt that you get on navigating to the above URL.
- Once successful authentication has occurred you will get a blank page.
- Now enter the URL to get domains in the same browser window:
http://youralmserver:port/qcbin/api/domains
- Once you enter this URL and press enter you will get domain list you have access to in XML format.
For ALM Versions prior to 12.53, in URLs, replace
api
withrest
e.g.
http://youralmserver:port/qcbin/rest/domains
Refer HP ALM REST API - Authentication for details on how to sign-in in older versions of ALM.
Sample Output
<Domains>
<Domain Name="Domain1" />
<Domain Name="Domain2" />
<Domain Name="Domain3" />
<Domain Name="Domain4" />
<Domain Name="Domain5" />
</Domains>
Code Method
For ALM Versions prior to 12.53, in the code replace
/api/domains
with/rest/domains
Parameters Required
Parameter | Example / Comments |
---|---|
HP ALM Base URL | http://youralmurl:port/qcbin |
Authentication CookieContainer | Pass the authentication cookie container obtained while you authenticated. Refer HP ALM REST API - Authentication for details. |
VB.Net Code to get ALM Domains
Private Function GetDomains(almBaseUrl As String, authenticationCookieContainer As CookieContainer) As IEnumerable(Of String)
Dim domainList = New List(Of String)()
Dim domainRequest = CType(WebRequest.Create(almBaseUrl + "/api/domains"), HttpWebRequest)
domainRequest.CookieContainer = authenticationCookieContainer
Dim domainResponse = domainRequest.GetResponse()
Dim domainResponseStream = domainResponse.GetResponseStream()
If domainResponseStream Is Nothing Then
Return domainList
End If
Using responseReader = New StreamReader(domainResponseStream)
Dim responseString = responseReader.ReadToEnd()
Dim responseXml = XElement.Parse(responseString)
Dim domainQuery = From domain In responseXml.Elements() Select New With {.domainName = domain.Attribute("Name").Value}
domainList.AddRange(domainQuery.[Select](Function(domain) domain.domainName))
End Using
Return domainList
End Function
Usage of VB.NET code
Dim almDomains = GetDomains("http://youralmurl:port/qcbin", AuthenticationCookieContainer)
Console.WriteLine(almDomains.Aggregate(Function(a, b) a + vbLf + b))
C# Code to get ALM Domains
private static IEnumerable GetDomains(string almBaseUrl, CookieContainer authenticationCookieContainer)
{
var domainList = new List();
var domainRequest = (HttpWebRequest)WebRequest.Create(almBaseUrl + "/api/domains");
domainRequest.CookieContainer = authenticationCookieContainer;
var domainResponse = domainRequest.GetResponse();
var domainResponseStream = domainResponse.GetResponseStream();
if (domainResponseStream == null) return domainList;
using (var responseReader = new StreamReader(domainResponseStream))
{
var responseString = responseReader.ReadToEnd();
var responseXml = XElement.Parse(responseString);
var domainQuery = from domain in responseXml.Elements()
select new { domainName = domain.Attribute("Name").Value };
domainList.AddRange(domainQuery.Select(domain => domain.domainName));
}
return domainList;
}
Usage of C# code
var almDomains = GetDomains("http://youralmurl:port/qcbin", authenticationCookieContainer);
Console.WriteLine(almDomains.Aggregate((a, b) => a + "\n" + b));
Output Sample
Domain1
Domain2
Domain3
Domain4
Domain5
- Programming
- Software Testing
Categories: