How to fix Date Time data type issue in Blue Prism?
. 4 mins read
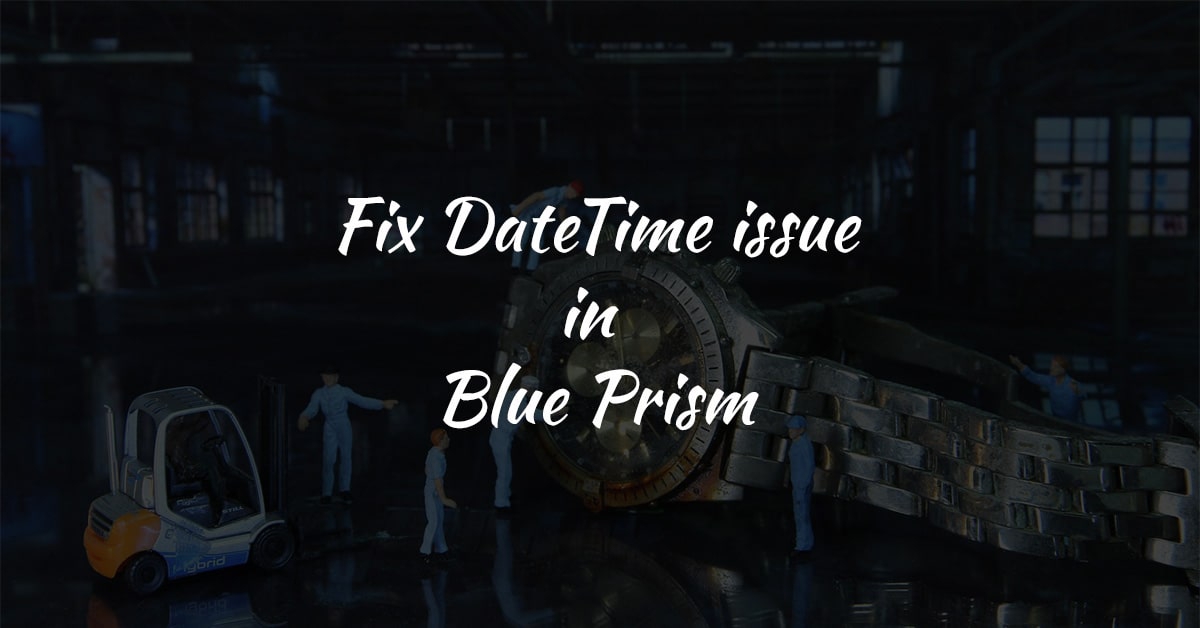
Wait, what? Is there an issue with Date Time data type in Blue Prism?
Unfortunately, yes. Blue Prism converts Date Time datatype returned by code stages to UTC without any consideration about user’s intention.
The Problem
In any automation project, we must deal with dates. We might have to manipulate dates, get dates from SharePoint etc. For some of these activities, we make use of code stage in Blue Prism.
To explain the problem of date time data type in Blue Prism, let us consider a scenario where we want to return a date time data type using a date time constructor.
Let us use the date time constructor as new DateTime(2012, 12, 25, 10, 30, 50);
.
This should return a date-time data type with value 25 December 2012 10:30:50
.
Now, let us use this in a Blue Prism code stage.
Blue Prism Code Stage
Input
For this example, we have a hardcoded date time constructor represented as new DateTime(2012, 12, 25, 10, 30, 50);
.
Outputs
Name | Data Type | Store In |
---|---|---|
dateFromCodeString | Text | String Representation of Date from Code |
bpDate | DateTime | Blue Prism Date Time Object |
The Code
DateTime dateFromCode = new DateTime(2012, 12, 25, 10, 30, 50);
dateFromCodeString = dateFromCode.ToString("dd-MM-yyyy HH:mm:ss");
bpDate = dateFromCode;
Analyzing Output
Name | Expected Value | Actual Value |
---|---|---|
String Representation of Date from Code | 25-12-2012 10:30:50 | 25-12-2012 10:30:50 |
Blue Prism Date Time Object | 25-12-2012 10:30:50 | 25-12-2012 05:00:50 |
The Difference
For the above example, we ran Blue Prism on a machine with the time zone configured as Indian Standard Time (IST). IST is 5 hours and 30 minutes ahead of UTC.
We can observe the same difference between the expected date time value and the actual date time value returned by Blue Prism.
Actual Value = Expected Value - 5 hours 30 minutes
25-12-2012 05:00:50 = (25-12-2012 10:30:50 - 05:30) = 25-12-2012 05:00:50
Problem Conclusion
From the example above, we can conclude that Blue Prism converts the date time data type returned from a code stage to UTC. Blue Prism does this conversion based on the local time zone of the machine in which it is running.
How to fix the problem?
To fix this problem, we need to understand what Blue Prism does to the actual date time object returned from the code stage.
If we take the above example, the local machine time zone is IST. IST has an offset of 5 hours and 30 minutes from UTC.
So, Blue Prism subtracted the offset from the date time returned by the code stage.
To fix this, we must add the offset back to the date returned from code stage. We can do this addition within the code stage itself.
In a way we are giving Blue Prism a date time with an extra offset added, so that when it converts to UTC, we get the desired date time.
Steps to fix
- Find the UTC offset of the local time.
var utcOffset = System.TimeZoneInfo.Local.GetUtcOffset(dateFromCode);
- Add the UTC offset to the date from code.
bpDate = dateFromCode.Add(utcOffset);
The final Code
DateTime dateFromCode = new DateTime(2012, 12, 25, 10, 30, 50);
dateFromCodeString = dateFromCode.ToString("dd-MM-yyyy HH:mm:ss");
var utcOffset = System.TimeZoneInfo.Local.GetUtcOffset(dateFromCode);
bpDate = dateFromCode.Add(utcOffset);
Analyzing the fixed Output
Name | Expected Value | Actual Value |
---|---|---|
String Representation of Date from Code | 25-12-2012 10:30:50 | 25-12-2012 10:30:50 |
Blue Prism Date Time Object | 25-12-2012 10:30:50 | 25-12-2012 10:30:50 |
Alternate Approach
If you know the time zone settings of the Blue Prism bot machine and you are sure that nobody will change the settings, you can add the timezone difference to the date time returned by Blue Prism to get the actual time. However, this is not a recommended method.
DateTime dateFromCode = new DateTime(2012, 12, 25, 10, 30, 50);
dateFromCodeString = dateFromCode.ToString("dd-MM-yyyy HH:mm:ss");
var timeSpanAdjustment = new System.TimeSpan(5, 30, 0);
bpDate = dateFromCode.Add(timeSpanAdjustment);
If your timezone is UTC - 3
use adjustment as new System.TimeSpan(-3, 0, 0);
.
If your timezone is UTC - 4:30
use adjustment as new System.TimeSpan(-4, 30, 0);
.
- RPA
Category:
- #Blue Prism
Tag: