Drag and drop items from windows explorer to application UFT
. 3 mins read
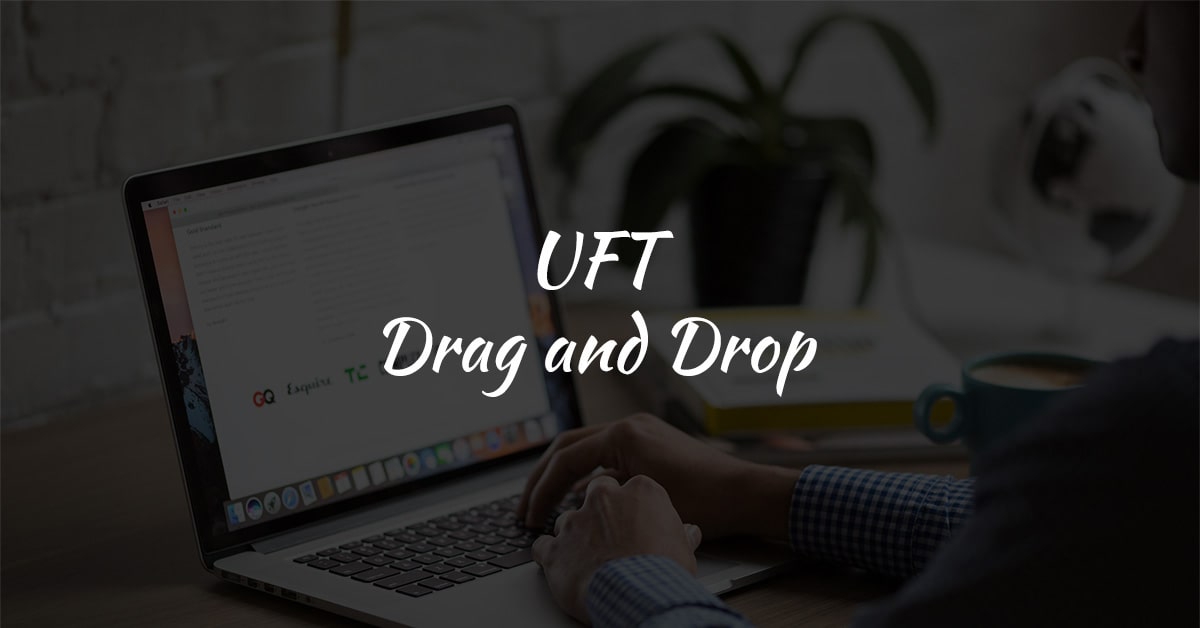
A tutorial on how to drag and drop items from windows explorer to an application using UFT/QTP.
QTP or UFT does not have a default functionality to drag an item from the windows explorer window to application under test.
Let us consider a scenario where we want to drag an image from windows explorer to TinEye, which is one of the best image search and recognition company.
Manual steps
- Access TinEye website.
- Identify the area where a drag and drop facility is available.
- Open image location using windows explorer.
- Select the image in the folder and drag it to the website.
If you try to record these steps with UFT, you will discover that UFT will not capture the drag and drop operation. This is because of the security features set up in Windows. For this to work in UFT you have to run UFT as administrator and run windows explorer as administrator programmatically. Windows security settings will not allow this to happen. In this post, we introduce a subroutine as a workaround for this problem.
Here is what the subroutine does.
Parameters to pass
- FilePath - the path of the file to drag and drop (e.g.
"C:\Users\Public\Pictures\Sample Pictures\Koala.jpg"
) - DropAreaObject - pass the object on which file has to be dropped
Subroutine Action
- Check whether the file exists
- Create a browser window object
- Create explorer windows object
- Open folder path
- Resize and move the folder window
- Select the item passed
- Detect the position of the file in the explorer window
- Resize and move the browser window
- Move the drop area to view
- Detect the position of the drop area
- Drag item from windows explorer to drop area and release mouse to drop the item
The Subroutine
Sub DragItemFromWindowsExplorerToIe(ByVal FilePath, ByVal DropAreaObject)
'Creating file system object
Set fso = CreateObject("Scripting.FileSystemObject")
'Checking if file exist
If fso.FileExists(FilePath) Then
'Creating device replay object
Set dr = CreateObject("Mercury.DeviceReplay")
'Creating Windows forms control object
Set ctlr = DotNetFactory.CreateInstance("System.Windows.Forms.Control")
FolderPath = fso.GetParentFolderName(FilePath)
FolderPathArray = Split(FolderPath, "\")
FolderName = FolderPathArray(UBound(FolderPathArray))
FileName = fso.GetFileName(FilePath)
'Adding description for Internet Explorer Window
Set IEWindowDesc = Description.Create
IEWindowDesc("micclass").Value = "Window"
IEWindowDesc("regexpwndtitle").Value = ".*Internet Explorer.*"
IEWindowDesc("regexpwndclass").Value = "IEFrame"
Set IEWindow = Window(IEWindowDesc)
'Adding description for Folder Window
Set ExplorerWindowDesc = Description.Create
ExplorerWindowDesc("micclass").Value = "Window"
ExplorerWindowDesc("regexpwndtitle").Value = FolderName
ExplorerWindowDesc("text").Value = FolderName
ExplorerWindowdesc("Location").Value = 0
ExplorerWindowDesc("regexpwndclass").Value = "CabinetWClass"
Set ExplorerWindow = Window(ExplorerWindowDesc)
'Opening the folder path
SystemUtil.Run FolderPath,"","","explore"
'Resizing and moving the folder window
ExplorerWindow.Resize 600,700
ExplorerWindow.Move 0,0
Set FileToDrag = ExplorerWindow.WinObject("nativeclass:=window", "acc_name:=Items View").WinList("nativeclass:=list", "acc_name:=Items View")
FileToDrag.Select FileName
FilePosX = ctlr.MousePosition.X
FilePosY = ctlr.MousePosition.Y
'Moving the browser window
IEWindow.Restore
IEWindow.Move 700, 0
DropAreaObject.Object.scrollIntoView
DropAreaX = DropAreaObject.GetROProperty("abs_x")
DropAreaY = DropAreaObject.GetROProperty("abs_y")
dr.DragAndDrop FilePosx, FilePosY, DropAreaX, DropAreaY, LEFT_MOUSE_BUTTON
Set dr = Nothing
Set ctlr = Nothing
End If
Set fso = Nothing
End Sub
Working Sample
SystemUtil.Run("https://tineye.com")
Wait(5)
Set DragArea = Browser("openurl:=https://tineye.com").Page("url:=https://tineye.com").WebEdit("type:=text", "name:=url", "html tag:=INPUT", "index:=0")
DragItemFromWindowsExplorerToIe "C:\Users\Public\Pictures\Sample Pictures\Koala.jpg", DragArea
- Software Testing
Category:
- #Automation Testing
- #UFT
Tags: