How to deserialize JSON in UiPath?
. 6 mins read
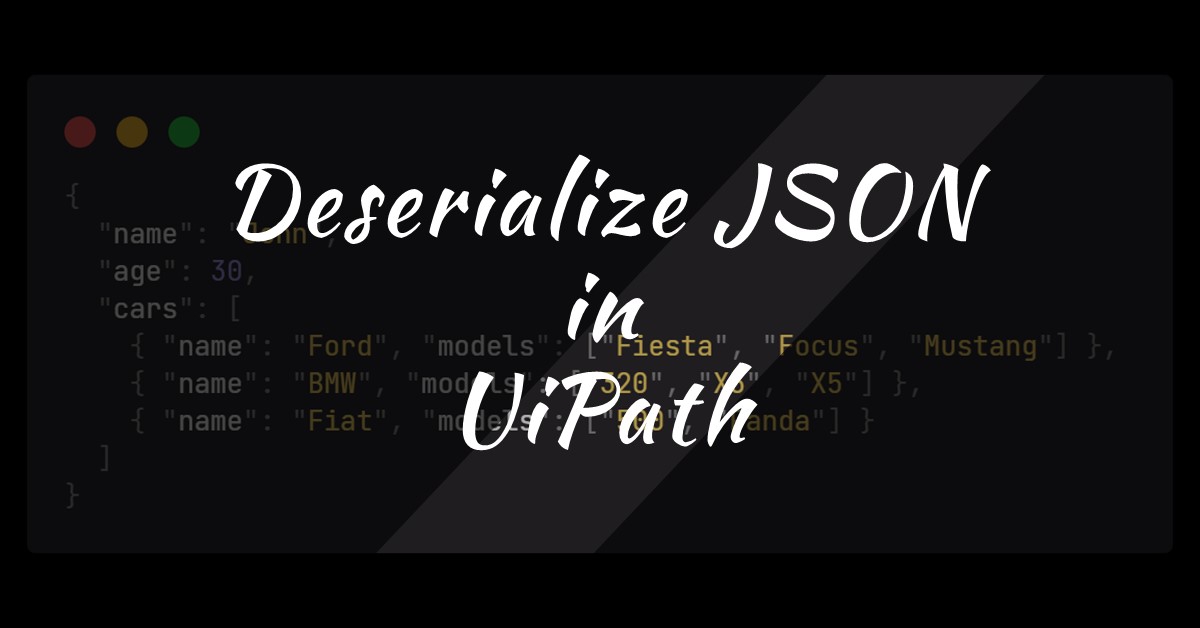
In this post, I will explain how to properly use the JSON activities in UiPath. We will cover in detail how to use the deserialize activities provided by UiPath. Before diving deep into UiPath activities for JSON we should understand what JSON is.
What is JSON?
Skip this section if you already have a good understanding of JSON.
JSON
stands for JavaScript Object Notation. It is an open-standard file format that uses human-readable data objects. A JSON string can contain key-value pairs and array data types. Because of its easy readability and ease of use, JSON has started service as a replacement for XML.
JSON Data Types
In JSON we can store values of the following data types
- string
- number
- another JSON object
- array
- boolean
- null
JSON Objects
- Curly braces
{}
surround a JSON Object. - JSON objects are represented as key-value pairs.
- All keys should be strings.
- Values could be any valid JSON data type.
- Key and values should be separated by a colon
:
. - Key-value pairs should be separated by a comma
,
.
Sample JSON Object
{
"site": "Aneejian",
"URL": "https://aneejian.com",
"postId": 32,
"liked": true
}
JSON Arrays
A JSON array is similar to arrays in other programming languages.
- Square brackets
[]
surround a JSON Array. - Array values could be any valid JSON data type .
- JSON array can store multiple data types.
- Values in a JSON Array should be separated by a comma
,
.
Sample JSON Array
[
"India",
"Australia",
"England"
]
Handling JSON in UiPath
Before getting started, we should set up UiPath.WebAPI.Activities
activity in your UiPath project. You can find the activity under the Official (
https://www.myget.org/F/workflow/
) feed of UiPath.
After installing you will be able to find the JSON activities from activities panel under Available » Programming » JSON
The JSON activities in UiPath is comprised of two sub-activities.
- Deserialize JSON to handle any JSON Object.
- Deserialize JSON Array to handle any JSON arrays.
Deserialize JSON Activity
Deserialize JSON Activity deserialize JSON object strings. Curly braces {}
surround a JSON object.
To explain this, let us consider the following JSON object string. We can save it in a file named jsonObject.json
{
"site": "Aneejian",
"URL": "https://aneejian.com",
"postId": 32,
"liked": true
}
Follow the steps below to read and deserialize the JSON Object string with UiPath. Save it to a .json
file.
- Read the JSON file using
Read Text File
activity and save the output to a variablejsonObjectString
. - Now pass the
jsonObjectString
toDeserialize JSON
Activity and save the output to a variable namedjsonObjectData
. Please note, the output data ofDeserialize JSON
Activity is aJObject
. - So, now we have a variable
jsonObjectData
of typeJObject
.
How to get values from a JObject?
We can use the following assign activities to get the values.
- To get
site
which is of data typestring
-jsonObjectData("site").ToString
- To get
url
which is again of datatypestring
-jsonObjectData.Item("url").ToString
- To get
postId
which is of typeinteger
-CInt(jsonObjectData.Item("postId"))
- To get
liked
whose datatype isBoolean
-Convert.ToBoolean(jsonObjectData("liked"))
With the above code, we have got individual values of all keys.
Deserialize JSON Array Activity
This activity deserialize JSON Array strings. JSON Arrays are surrounded by square brackets []
.
To explain this, let us consider the following JSON Array string. Save it to a .json
file.
[
"Raul",
"Jasmine",
"Susan"
]
Follow the steps below to read and deserialize the JSON Array string with UiPath.
- Read the JSON file using
Read Text File
activity and save the output to a variablejsonArrayString
. - Now pass the
jsonArrayString
toDeserialize JSON Array
activity and save the output to a variable namedjsonArrayData
. - Please note, the output data of
Deserialize JSON Array
activity is aJArray
. So, now we have a variablejsonArrayData
of typeJArray
.
How to get values from a JArray?
There are multiple ways by which we can retrieve data from a JArray
.
- A
For Each
loop can iterate through each item in aJArray
. - Get items by index. -
jsonArrayData.Item(2)
will returnSusan
.
Deserializing a complex JSON
Now let us see how to deserialize a mixed JSON with both JSON Objects and JSON Arrays in it.
Sample JSON
{
"name":"John",
"age":30,
"cars": [
{ "name":"Ford", "models":[ "Fiesta", "Focus", "Mustang" ] },
{ "name":"BMW", "models":[ "320", "X3", "X5" ] },
{ "name":"Fiat", "models":[ "500", "Panda" ] }
]
}
Once we get a JSON string to parse, we need to understand its structure.
Let us say, we got the above JSON string from a website which represents car wishlist of their customers. From UiPath, we have to convert it to the following string.
John, aged 30 has shortlisted 8 cars from 3 different brands.
The cars shortlisted are Ford Fiesta, Ford Focus, Ford Mustang, BMW 320, BMW X3, BMW X5, Fiat 500, and Fiat Panda.
To form the string above we need to identify the following parameters.
- Name of the person.
- Age of the person.
- Cars array.
- The number of brands he shortlisted.
- The number of cars the person shortlisted.- This would be the sum of all models under each brand the person has shortlisted.
- Name of each car with brand name prefixed.
Let us see how we can do this.
Step 1: Deserialize the base JSON string
Since the string is surrounded by curly braces {}
, it represents a JSON object. So, we have to use Deserialize JSON
activity on the string.
After using the deserialize activity, we will get the values of the following parameters. Let us assume we saved the output of activity into a variable named personData
.
- Person name by using
personData("name").ToString
- Age of the person by using
personData("age").ToString
- Cars array by using
personData("cars").ToString
Step 2: Deserialize the cars string
Since the cars string is surrounded by square brackets []
, it represents a JSON Array. So, we have to use Deserialize JSON Array
activity on the string.
After activity execution, we will have values of the following parameters.
- Number of brands by using
carsArray.Count
- By iterating through the
carsArray
we can get details of the cars also. For that, we have to useDeserialize JSON
on each item.
Wait, is it that complex?
Actually not. You can get values of all the parameters we require just by using the deserialization done at the first step.
Here is how.
We can find the number of car brands by using personData("cars").Count.ToString
So, we can directly access the JArray
object by using personData("cars")
.
If we go further and use personData("cars").Item(0)("models").Count
we will get the number of Ford
cars.
I believe you got the idea. Do the rest as an assignment. Please comment if you have any doubts or queries.